FPSゲームでは、アイテムや武器を拾うシステムは必要ですよね。
今回はそんなシステムの作り方を紹介していこうと思います。
準備
実験ように武器を三つ用意
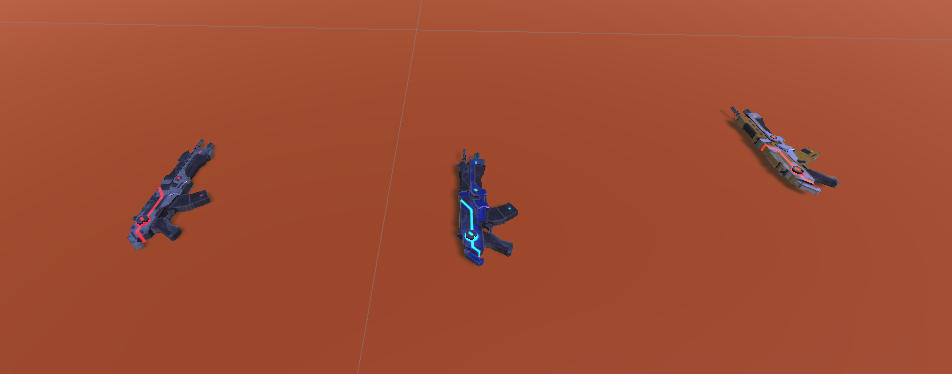
また、武器には「Box Collider」と「Rigidbody」がなければレイキャストが識別できないので注意。
拾うことができるオブジェクトにはタグをつけます。

次にプレイヤーを用意します。
こちらのアセットを使用してプレイヤーを用意します↓
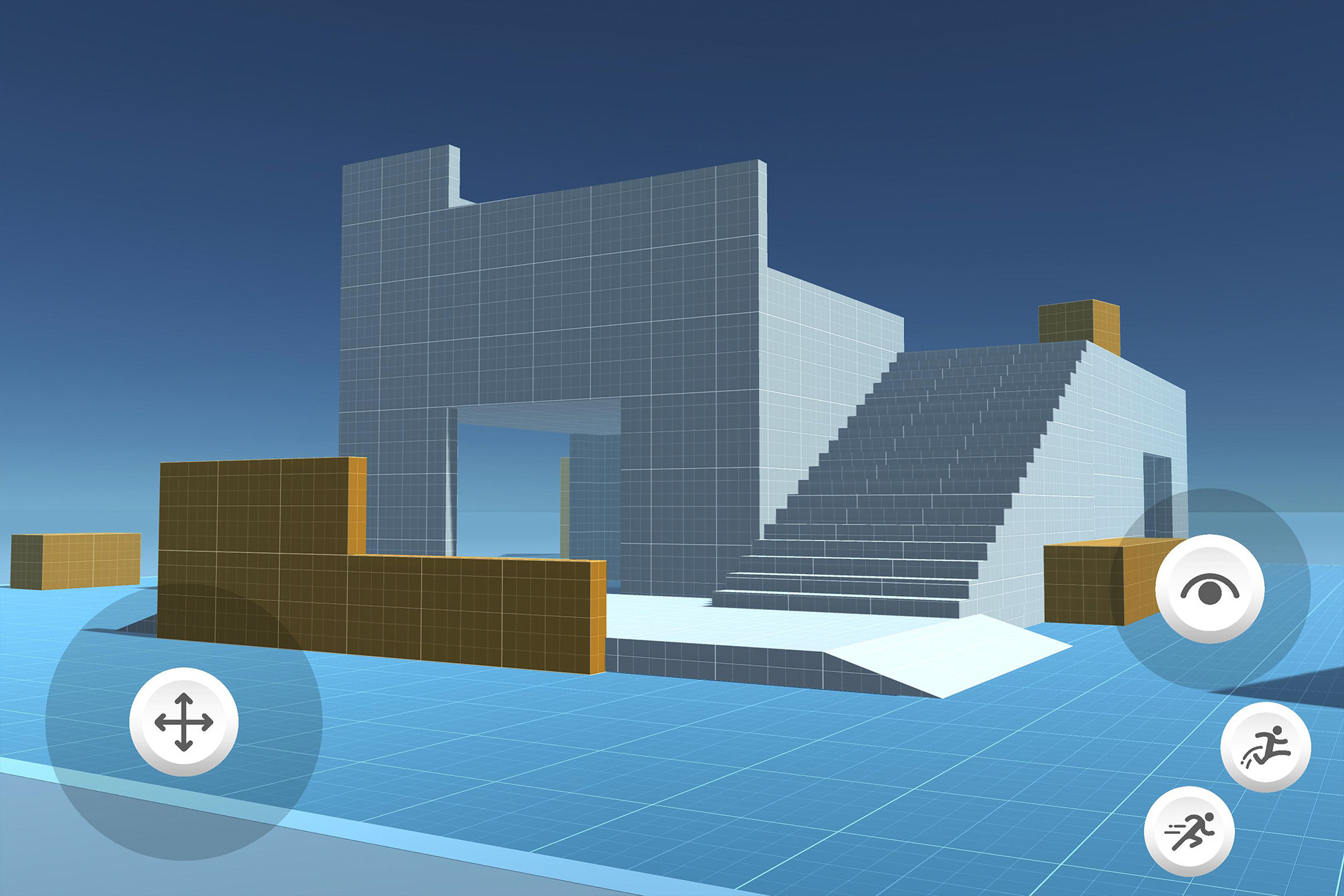
Starter Assets - FirstPerson | Updates in new CharacterController package | Essentials | Unity Asset Store
Get the Starter Assets - FirstPerson | Updates in new CharacterController package package from Unity Technologies and sp...
プレイヤーを用意したら武器の位置を固定するための空のオブジェクトを用意。
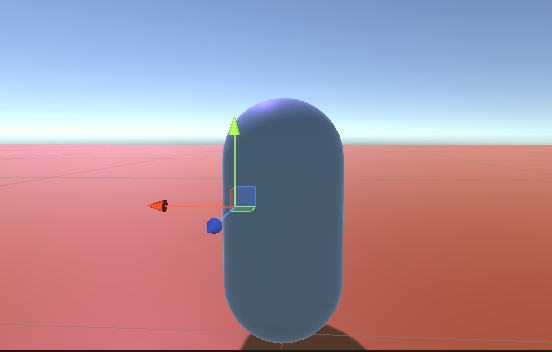
この空のオブジェクトはMain Cameraの子オブジェクトにします。
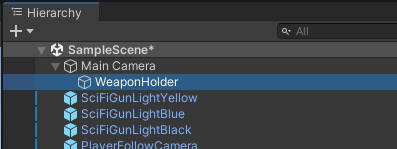
このオブジェクトは、武器を拾った時の位置になるので必要です。
これで準備は完了です。
拾うためのスクリプト
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class WeaponPickUp : MonoBehaviour
{
public Transform equipePosition;
public float distance = 30f;
GameObject currentWeapon;
GameObject wp;
bool canGrab;
void Start()
{
}
// Update is called once per frame
void Update()
{
checkWeapons();
if (canGrab)
{
if (Input.GetKeyDown(KeyCode.E))
{
if (currentWeapon != null)
Drop();
PickUp();
}
}
if (currentWeapon != null)
{
if (Input.GetKeyDown(KeyCode.Q))
{
Drop();
}
}
}
private void checkWeapons()
{
RaycastHit hit;
if (Physics.Raycast(Camera.main.transform.position, Camera.main.transform.forward, out hit, distance))
{
if (hit.transform.tag == "CanGrab")
{
canGrab = true;
wp = hit.transform.gameObject;
}
}
else
{
canGrab = false;
}
}
private void PickUp()
{
currentWeapon = wp;
currentWeapon.transform.position = equipePosition.position;
currentWeapon.transform.parent = equipePosition;
currentWeapon.transform.localEulerAngles = new Vector3(0, 0, 0);
currentWeapon.GetComponent<Rigidbody>().isKinematic = true;
currentWeapon.GetComponent<BoxCollider>().enabled = false;
}
private void Drop()
{
currentWeapon.transform.parent = null;
currentWeapon.GetComponent<Rigidbody>().isKinematic = false;
currentWeapon.GetComponent<BoxCollider>().enabled = true;
currentWeapon = null;
}
}
これで「Eを押して拾う、Qを押してドロップ」することができます。
また、現在所持している武器をドロップし、別の武器を拾うこともできます。
このスクリプトをプレイヤーにアタッチします。
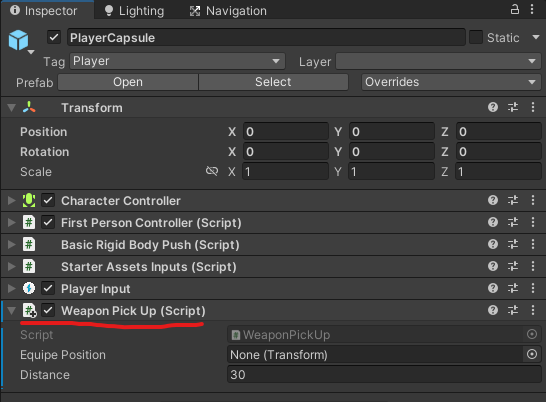
Equipe Positionという項目がありますので、そこに先ほど作った武器の位置を固定する空のオブジェクトをアタッチしましょう。
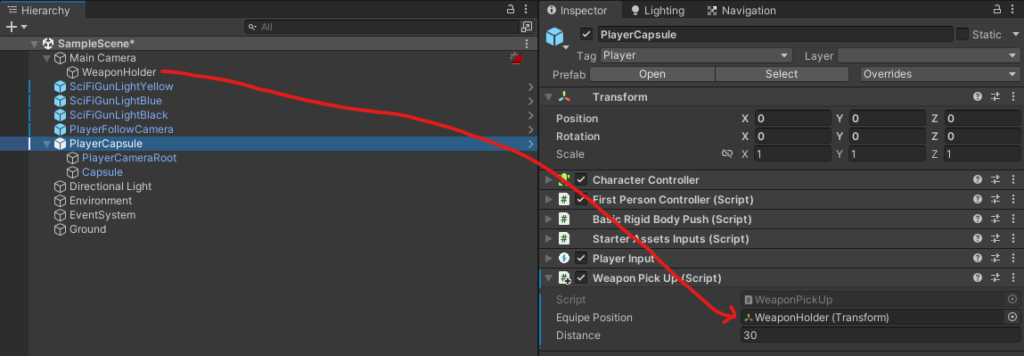
これで実行をしてみましょう。
完成です!
まとめ
こんな感じでレイキャストはFPSにおけるアイテムや武器との接触に必要なものになっているのでぜひ活用しましょう。
コメント